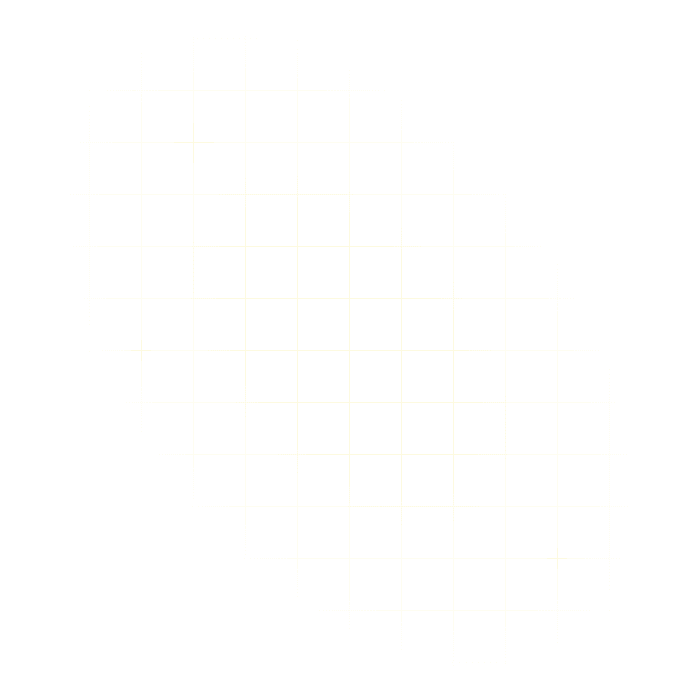
API Advice
Type Faith vs Type Safe

Sagar Batchu
April 16, 2024

Type Faith vs Type Safe
Introduction
Type safety is a core aspect of modern programming, ensuring that errors related to mismatched or incorrect data types are caught at compile time rather than during execution. The evolution of JavaScript—a language known for its flexibility and lack of strict type enforcement—saw a significant shift with the introduction of TypeScript. TypeScript, developed by Microsoft, brought static type checking to the JavaScript ecosystem, helping developers catch errors early in the development process.
However, TypeScript isn’t without its limitations. While it enforces type safety within the codebase, it cannot guarantee the correctness of data coming from external sources like APIs. This becomes a critical issue when developers assert incorrect data types received from APIs, leading to potential runtime errors and system failures.
The good news for API builders is that it is now possible to provide end-to-end type safety in their libraries, ensuring that data types are consistent throughout the application and API interactions.
Type Faith
Developers often opt out of implementing end-to-end type safety in their libraries due to the complexity and additional coding required. This decision places a significant burden on end users who must then ensure data type correctness in their applications, a process that is both error-prone and time-consuming.
For example, consider a scenario where an API is expected to return a list of user objects, but due to changes in the backend, it starts returning mixed types (users and admin objects). A client application relying on type faith—assuming the returned data matches the expected types without validation—may perform operations that are valid for user objects but not for admin objects, leading to runtime errors and application crashes.
Type Safe
To achieve true type safety, developers can use tools like Zod to validate and enforce data types at runtime. Zod allows for the definition of schemas that describe the expected shape and type of data. Here’s a simple example of how Zod can be used to enforce type safety when handling API responses
import { z } from 'zod';// Define a schema for the user objectconst UserSchema = z.object({id: z.number(),name: z.string(),email: z.string().email(),});// Function to validate API responsefunction validateApiResponse(data: unknown) {try {// Validate data against the schemaUserSchema.parse(data);console.log('Data is valid!');} catch (error) {console.error('Invalid data:', error);}}// Simulated API responseconst apiResponse = {id: 1,name: "John Doe",email: "john@example.com",};validateApiResponse(apiResponse);
Using such validations, the end developer who consumes the API can trust that the data they are working with is correctly typed, reducing the risk of bugs and improving the reliability of the application.
Conclusion
Implementing end-to-end type safety requires additional effort from the developers building the API libraries. However, the benefits in terms of application stability, security, and developer productivity are immense. As the ecosystem evolves, the tools and practices around type safety continue to improve, making it increasingly feasible to achieve robust type safety across the board. By adopting these practices, developers can ensure that their applications are not only functional but also secure and resilient against type-related errors.