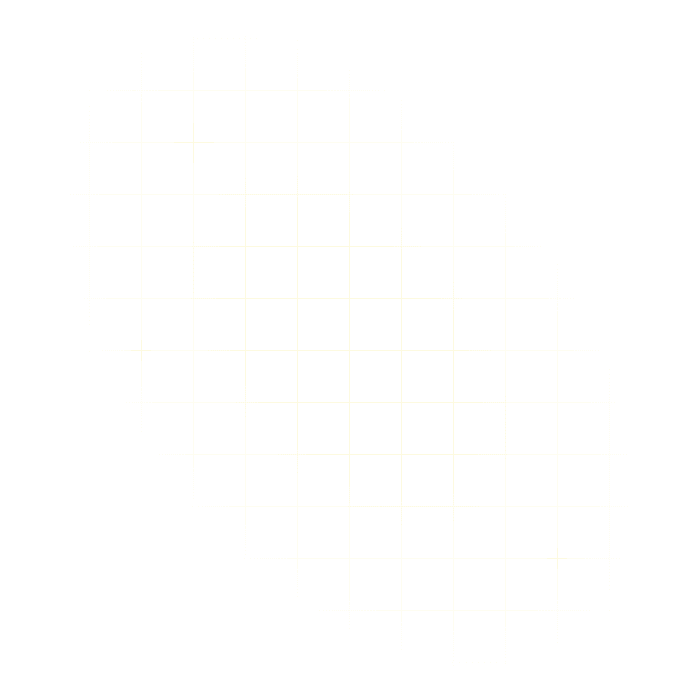
Product Updates
Python Generation with Async & Pydantic Support

Tristan Cartledge
July 24, 2024

Beta Availability
Now in beta! All new Python SDKs will use the new generator by default. If you’d like to try it upgrade an existing Python SDK in production, check out our migration guide.
Today, we’re announcing the release of our new Python Generator! The new generator takes full advantage of the best tooling available in the Python ecosystem to introduce true end-to-end type safety, support for asynchronous operations, and a streamlined developer experience.
The full details are below, but here are the headline features that come included in the new Python SDKs:
- Full type safety with Pydantic (opens in a new tab) models for all request and response objects.
- Support for both asynchronous and synchronous method calls using
HTTPX
. - Support for typed dicts as method inputs for an ergonomic interface.
Poetry
for dependency management and packaging.- Improved IDE compatibility for a better type checking experience.
- A DRYer and more maintainable internal library codebase.
And if you want to see new SDKs in the wild, check out the SDK from our design partner:
End-to-end Type Safety with Pydantic
Pydantic is a data modeling library beloved in the Python ecosystem. It enhances Python’s type hinting annotations, allowing for more explicit API contracts and runtime validation.
And now, Speakeasy generates Pydantic models for all request and response objects defined in your API. The request models ensure that your user’s data is correct at run time, while the response models validate the data returned by the server matches the contract.
The Pydantic-powered hints and error messages presented to users helps them ensure identify and correct errors before they cause issues downstream. This functionality is crucial for maintaining data integrity and reliability in applications that rely on your APIs.
Enhanced Asynchronous and Synchronous Support
import beezy_aifrom beezy_ai import BeezyAIimport oss = BeezyAI(api_key_auth=os.getenv("API_KEY"),)res = s.chat.stream(request={"model": "beezy-small-latest","messages": [{"role": beezy_ai.ChatCompletionRole.USER,"content": "What's the difference between OpenAPI and Swagger??",},],"max_tokens": 512,})if res is not None:for event in res:# handle eventprint(event)
import asyncioimport beezy_aifrom beezy_ai import BeezyAIimport osasync def main():s = BeezyAI(api_key_auth=os.getenv("API_KEY"),)res = await s.chat.stream_async(request={"model": "beezy-small-latest","messages": [{"role": beezy_ai.ChatCompletionRole.USER,"content": "What's the difference between OpenAPI and Swagger??",},],"max_tokens": 512,})if res is not None:for event in res:print(event)asyncio.run(main())
As the Python ecosystem has expanded to support data intensive, real-time applications, asynchronous support has grown in importance. That’s why we’ve built our Python SDKs on top of HTTPX
. Python SDKs will now support both asynchronous and synchronous method calls.
And to make it as ergonomic as possible, there’s no need for users to declare separate sync & async clients if they need both. Just instantiate one SDK instance and call methods sync or async as needed.
Support for TypedDict
Input
from clerk_dev import Clerks = Clerk()res = s.invitations.create(email_address="user@example.com",ignore_existing=True, notify=True, public_metadata={},redirect_url="https://example.com/welcome")if res is not None:# handle responsepass
Continuing with our efforts to make the SDKs as ergonomic as possible. SDKs now support the use of TypedDict
s for passing data into methods. This feature allows you to construct request objects by simply listing off key: value
pairs.
The SDK will handle the construction of the request object behind the scenes. Just another way we’re making it easier for your users to get integrated with your APIs.
A Streamlined Developer Experience
It’s what on the inside that matters, so we’ve made significant improvements to the internal library code as well:
-
Improved Import Patterns: By refining how we handle imports internally, developers will see a more stable and predictable behavior in their IDE’s type hinting. This change helps maintain a cleaner namespace and reduces the chance of conflicts or errors due to improper imports.
-
Enhanced Dependency Management: Transitioning from
pip
topoetry
has streamlined our SDK’s setup and dependency management, making it easier to maintain and update.poetry
provides a more declarative way of managing project dependencies, which includes automatic resolution of conflicts and simpler packaging and distribution processes. -
Renamed Packages: To further enhance usability, we’ve decoupled SDK class names from package names, allowing for more intuitive and flexible naming conventions. This adjustment allows better organization and integration within larger projects, where namespace management is crucial.
-
DRYer Codebase: We’ve refactored our internal library code to reduce redundancy and improve code reuse. This makes it easier for users to step through the codebase and understand how the SDK functions.
These changes collectively reduce the complexity and increase the maintainability of projects using our SDK.
Looking Forward
The new Python Generator is just the beginning. We plan to continue refining the SDK based on user feedback. Over the next few weeks we’ll be moving to make this new generation the default for all new Python SDKs generated on the platform.
We are excited to see how the community puts these new features to work. Your feedback is invaluable to us, and we welcome everyone to join us in refining this tool to better suit the needs of the Python community.